Spring DI
: 설정파일 또는 어노테이션을 통해서 객체간의 의존관계를 설정할 수 있다. 따라서 객체는 의존하고 있는 객체를 직접 생성하거나 검색할 필요가 없다.
DI 용어정리
빈
- 스프링이 IoC방식으로 관리하는 오브젝트, 스프링이 직접 생성와 제어를 담당한다.
빈 팩토리(Bean Factory)
- 스프링이 IoC를 담당하는 핵심 컨테이너로, Bean을 등록, 생성, 조회, 반환하는 기능을 담당한다.
- 일반적으로 BeanFactory를 바로 사용하지 않고 이를 확장한 ApplicationContext를 이용한다.
애플리케이션 컨텍스트(Application Context)
- BeanFactory를 확장한 IoC컨테이너로, Bean을 등록하고 관리하는 기본적인 기능은 BeanFactory와 동일하다.
- 스프링이 제공하는 각종 부가서비스를 추가로 제공한다.
- BeanFactory라고 부를 때에는 주로 빈의 생성과 제어의 관점에서 이야기하는 것이고, Application Context라고 할 때는 스프링이 제공하는 애플리케이션 지원기능을 모두 포함해서 이야기 하는것으로 간주한다.
설정정보/설정메타정보(Configuration metadata)
- 스프링의 설정정보란 ApplicationContext 또는 BeanFactory가 IoC를 적용하기 위해 사용하는 메타정보를 말한다.
- 설정정보는 IoC컨테이너에 의해 관리되는 Bean객체를 생성하고 구성할때 사용된다.
스프링 프레임워크
- 스프링 프레임워크는 IoC컨테이너, Application Context를 포함해서 스프링이 제공하는 모든기능을 통틀어 말할 때 주로 사용
빈 생성범위
<bean id="~~" class="~~~~" scope="prototype">과 같이 지정해준다.
범위 | 설명 |
singleton | 기본값, 스프링 컨테이너당 하나의 인스턴스 빈만 생성 |
prototype | 컨테이너에 빈을 요청할 때 마다 새로운 인스턴스 생성 |
request | HTTP Request별 새로운 인스턴스 생성 |
session | HTTP Session별 새로운 인스턴스 생성 |
스프링 빈 설정 방법
XML
- xml문서 형대로 빈의 설정메타 정보 기술
- 단순하며 사용하기 쉽고, 가장많이 사용하는 방식이다
- <bean>태그를 통해 세밀한 제어 가능
ex) <bean id="userDao" class="com.test.hello.service.UserServiceImpl" scope="prototype">
<property name="UserDao" ref="UserDao"/>
</bean>
Annotation
- 어플리케이션의 규모가 커지고 빈의 개수가 많아질 경우 XML파일을 관리하는 것이 번거로움
- 빈으로 사용될 클래스에 annotation을 부여해주면 자동으로 빈 등록 가능
- annotation으로 빈을 설정할 경우 반드시 component-scan을 설정해야 한다
Stereotype | 적용대상 |
@Repository | Data Access Layer의 DAO 또는 Repository클래스에 사용 DataAccessException 자동변환과 같은 AOP의 적용대상을 선정하기 위해 사용 |
@Service | Service Layer의 클래스에 사용 |
@Controller | Presentation Layer의 MVC Controller에 사용 스프링 웹 서블릿에 의해 웹 요청을 처리하는 컨트롤러 빈으로 설정 |
@Component | 위의 Layer 구분을 적용하기 어려운 일반적인 경우에 설정 |
Spring Framework기반 도서정보 관리 애플리케이션
요구사항
- spring legacy 기반의 프로젝트
- springframework 버전 5 이상, JRE버전 1.8
- junit 버전 4.13.
- 기본 logger의 레벨 : debug
- interfacee에 선언된 메서드의 구현은 자동생성되는 코드로 구현
- UserServiceImpl은 UserRepo를 has a 관계로 포함하는데 생성자를 통해서 주입받는다.
- BookServiceImpl은 BookRepo를 has a 관계로 포함하는데 setter메서드를 통해 주입받는다
- 프로젝트 구조
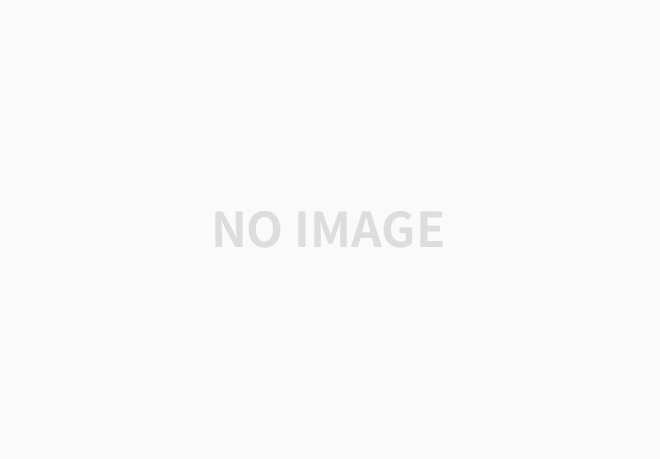
DTO
Book.java
package com.ssafy.ws.dto;
import java.io.Serializable;
public class Book implements Serializable{
private String isbn;
private String title;
private String author;
private int price;
private String content;
private String image;
public Book() {
super();
}
public Book(String isbn, String title, String author, int price, String content, String image) {
super();
this.isbn = isbn;
this.title = title;
this.author = author;
this.price = price;
this.content = content;
this.image = image;
}
public String getIsbn() {
return isbn;
}
public void setIsbn(String isbn) {
this.isbn = isbn;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
public String getImage() {
return image;
}
public void setImage(String image) {
this.image = image;
}
@Override
public String toString() {
return "Book [isbn=" + isbn + ", title=" + title + ", author=" + author + ", price=" + price + ", content="
+ content + ", image=" + image + "]";
}
}
User.java
package com.ssafy.ws.dto;
import java.io.Serializable;
public class User implements Serializable{
private String id;
private String name;
private String pass;
private String rec_id;
public User() {
super();
}
public User(String id, String name, String pass, String rec_id) {
super();
this.id = id;
this.name = name;
this.pass = pass;
this.rec_id = rec_id;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPass() {
return pass;
}
public void setPass(String pass) {
this.pass = pass;
}
public String getRec_id() {
return rec_id;
}
public void setRec_id(String rec_id) {
this.rec_id = rec_id;
}
@Override
public String toString() {
return "User [id=" + id + ", name=" + name + ", pass=" + pass + ", rec_id=" + rec_id + "]";
}
}
repo
BookRepo.java
package com.ssafy.ws.model.repo;
import java.util.List;
import com.ssafy.ws.dto.Book;
public interface BookRepo {
int insert(Book book);
int update(Book book);
int delete(String isbn);
Book select(String isbn);
List<Book> search();
}
BookRepoImpl.java
package com.ssafy.ws.model.repo;
import java.util.List;
import org.springframework.stereotype.Repository;
import com.ssafy.ws.dto.Book;
@Repository
public class BookRepoImpl implements BookRepo {
@Override
public int insert(Book book) {
// TODO Auto-generated method stub
return 0;
}
@Override
public int update(Book book) {
// TODO Auto-generated method stub
return 0;
}
@Override
public int delete(String isbn) {
// TODO Auto-generated method stub
return 0;
}
@Override
public Book select(String isbn) {
// TODO Auto-generated method stub
return null;
}
@Override
public List<Book> search() {
// TODO Auto-generated method stub
return null;
}
}
UserRepo.java
package com.ssafy.ws.model.repo;
import com.ssafy.ws.dto.User;
public interface UserRepo {
User select(String id);
}
UserRepoImpl.java
package com.ssafy.ws.model.repo;
import org.springframework.stereotype.Repository;
import com.ssafy.ws.dto.User;
@Repository
public class UserRepoImpl implements UserRepo{
@Override
public User select(String id) {
// TODO Auto-generated method stub
return null;
}
}
service
BookService.java
package com.ssafy.ws.model.service;
import java.util.List;
import com.ssafy.ws.dto.Book;
public interface BookService {
int insert(Book book);
int update(Book book);
int delete(String isbn);
Book select(String isbn);
List<Book> search();
}
BookServiceImpl.java
package com.ssafy.ws.model.service;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.ssafy.ws.dto.Book;
import com.ssafy.ws.model.repo.BookRepo;
/**
* 빈으로 등록될 수 있도록 @Service를 선언한다.
*/
@Service
public class BookServiceImpl implements BookService {
/**
* has a 관계로 사용할 BookRepo 타입의 repo를 선언한다.
*/
private BookRepo repo;
/**
* setter를 통해 BookRepo를 주입받는다.
* @Autowired를 통해 BookRepo 타입의 빈을 주입 받는다.
* @param repo
*/
@Autowired
public void setBookRepo(BookRepo repo) {
this.repo = repo;
}
public BookRepo getBookRepo() {
return repo;
}
@Override
public int insert(Book book) {
// TODO Auto-generated method stub
return 0;
}
@Override
public int update(Book book) {
// TODO Auto-generated method stub
return 0;
}
@Override
public int delete(String isbn) {
// TODO Auto-generated method stub
return 0;
}
@Override
public Book select(String isbn) {
// TODO Auto-generated method stub
return null;
}
@Override
public List<Book> search() {
// TODO Auto-generated method stub
return null;
}
}
UserService.java
package com.ssafy.ws.model.service;
import com.ssafy.ws.dto.User;
public interface UserService {
User select(String id);
}
UserServiceImpl.java
package com.ssafy.ws.model.service;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.ssafy.ws.dto.User;
import com.ssafy.ws.model.repo.UserRepo;
/**
* 빈으로 등록될 수 있도록 @Service를 선언한다.
*
*/
@Service
public class UserServiceImpl implements UserService {
/**
* has a 관계로 사용할 UserRepo 타입의 repo를 선언한다.
*/
private UserRepo repo;
/**
* 생성자를 통해 UserRepo를 주입받는다.
* UserRepo 타입의 빈을 주입받기 위해 @Autowired를 사용한다.
* @param repo
*/
@Autowired
public UserServiceImpl(UserRepo repo) {
this.repo = repo;
}
@Override
public User select(String id) {
// TODO Auto-generated method stub
return null;
}
}
설정파일
application.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd">
<!-- com.ssafy.ws.model 패키지를 스캔해서 빈을 등록한다. -->
<context:component-scan base-package="com.ssafy.ws.model" />
<bean id="ds" class="org.apache.commons.dbcp2.BasicDataSource">
<property name="driverClassName" value="~~~~"></property>
<property name="url" value="~~~~"></property>
<property name="username" value="~~~~"></property>
<property name="password" value="~~~~"></property>
</bean>
</beans>
log4j.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE log4j:configuration PUBLIC "-//APACHE//DTD LOG4J 1.2//EN" "log4j.dtd">
<log4j:configuration xmlns:log4j="http://jakarta.apache.org/log4j/">
<!-- Appenders -->
<appender name="console" class="org.apache.log4j.ConsoleAppender">
<param name="Target" value="System.out" />
<layout class="org.apache.log4j.PatternLayout">
<param name="ConversionPattern" value="%-5p: %c - %m%n" />
</layout>
</appender>
<!-- Application Loggers -->
<logger name="com.ssafy.ws">
<level value="debug" />
</logger>
<!-- 3rdparty Loggers -->
<logger name="org.springframework.core">
<level value="info" />
</logger>
<logger name="org.springframework.beans">
<level value="info" />
</logger>
<logger name="org.springframework.context">
<level value="info" />
</logger>
<logger name="org.springframework.web">
<level value="info" />
</logger>
<!-- Root Logger -->
<root>
<priority value="warn" />
<appender-ref ref="console" />
</root>
</log4j:configuration>
'WEB > Spring' 카테고리의 다른 글
[Spring] Spring FileUpload + 예제 실습 (0) | 2022.04.24 |
---|---|
[Spring] Spring Interceptor /Filter + 예제 실습 (0) | 2022.04.24 |
[Spring] Spring AOP + 예제 실습 (0) | 2022.04.24 |
[Spring] Spring MVC + 예제 실습 (0) | 2022.04.23 |
[Spring] Spring이란 (0) | 2022.04.16 |
댓글